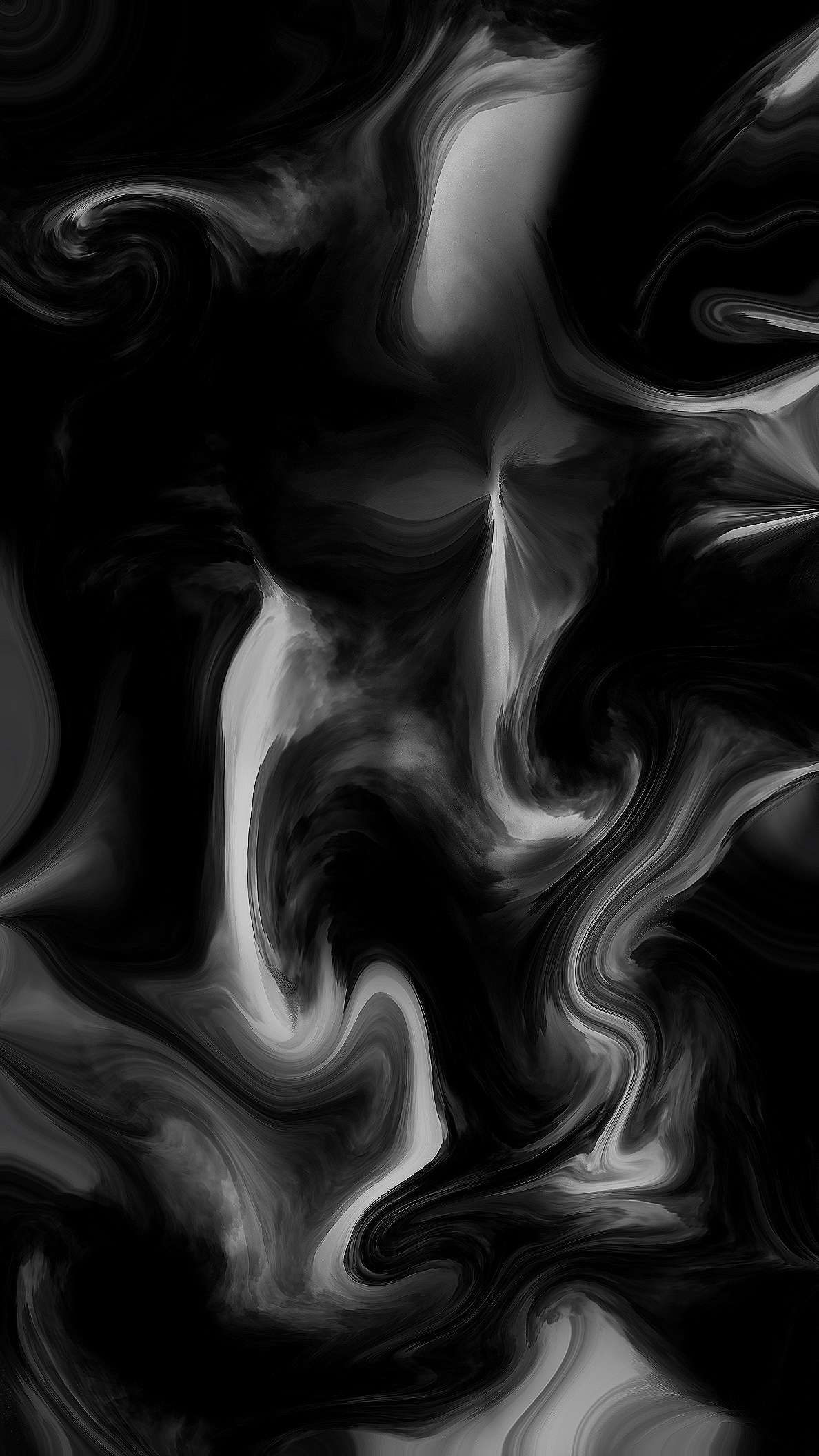
Pseudocode and Flowcharts
Assignment
Assignment Values are assigned to an item/variable using the ← operator. The variable on the left of the ← is assigned the value of the expression on the right
Conditional statements
A condition that can be true or false: IF … THEN … ELSE … ENDIF
Example: IF Age < 18
THEN PRINT “Child”
ELSE PRINT “Adult”
ENDIF
A choice between several different values: CASE … OF … OTHERWISE … ENDCASE, for example
CASE Grade OF
“A”: PRINT “Excellent”
“B”: PRINT “Good”
“C”: PRINT “Average”
OTHERWISE PRINT “Improvement is needed”
ENDCASE
IF … THEN … ELSE … ENDIF
For an IF condition the THEN path is followed if the condition is true and the ELSE path is followed if the condition is false. There may or may not be an ELSE path. The end of the statement is shown by ENDIF.
Example: IF Found
THEN PRINT “Your search was successful”
ELSE PRINT “Your search was unsuccessful”
ENDIF
CASE … OF … OTHERWISE … ENDCASE
For a CASE condition the value of the variable decides the path to be taken. Several values are usually specified. OTHERWISE is the path taken for all other values. The end of the statement is shown by ENDCASE.
Example: CASE Choice OF
1 : Answer ← Num1 + Num2
2 : Answer ← Num1 – Num2
3 : Answer ← Num1 * Num2
4 : Answer ← Num1 / Num2
OTHERWISE PRINT “Please enter a valid choice”
ENDCASE
Loop structures
When some actions performed as part of an algorithm need repeating, this is called ‘iteration’. Loop structures are used to perform the iteration.
FOR … TO … NEXT
FOR Counter ← 1 TO 10
PRINT “*”
NEXT Counter ← 0
REPEAT … UNTIL
REPEAT
PRINT “*”
Counter ← Counter + 1
UNTIL Counter > 10
WHILE … DO … ENDWHILE
Counter ← 0
WHILE Counter < 10 DO
PRINT “*”
Counter ← Counter + 1
ENDWHILE
Input and output statements
INPUT and OUTPUT are used for the entry of data and display of information
INPUT is used for data entry. It is usually followed by a variable where the data input is stored, for example:
INPUT Name
INPUT StudentMark
OUPUT/PRINT is used to display information either on a screen or printed on paper.
PRINT Name
PRINT “Your name is”, Name
OUTPUT Name1, Name2, Name3
Standard flowchart symbols
Flowcharts are drawn using standard symbols.
Begin/End
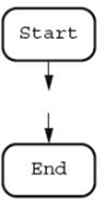
Process
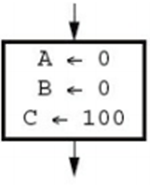
Input/Output
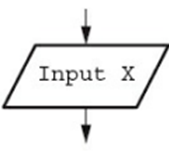
Decision
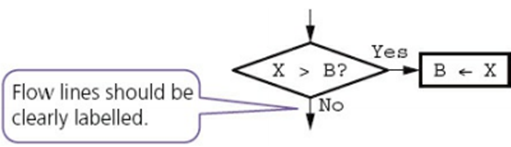
Flow lines
