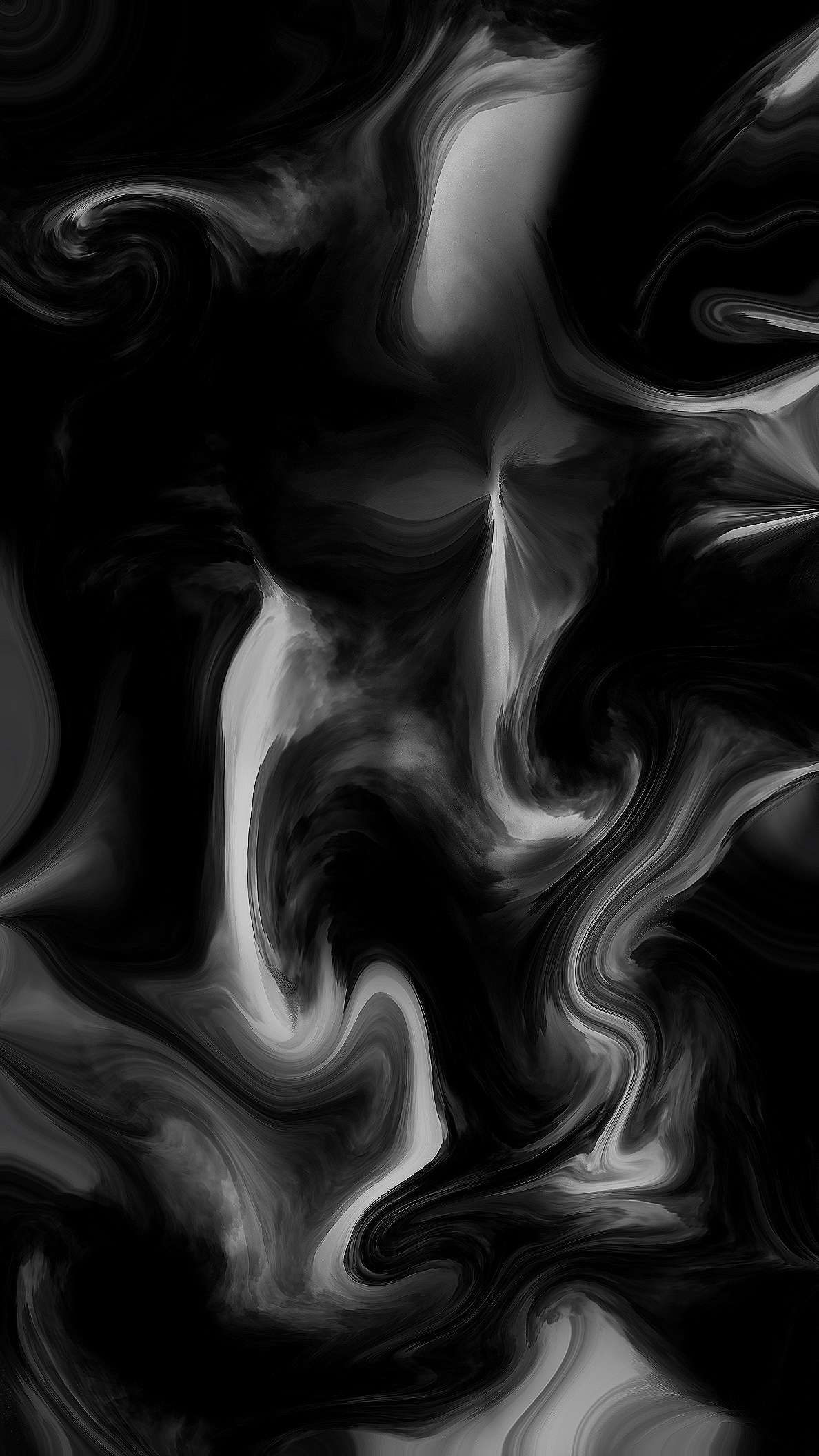
Programming Concepts
Programming
Programing languages:
• Scratch: a good basic introduction to programming for beginners that is freely available from MIT. Scratch makes programming easy and fun to learn as it uses visual building blocks. It is useful as an introduction.
• JavaScript: a scripting language that works in any browser, no download needed. JavaScript works with the HTML (see Section 1.6.2).
• Python: a general purpose, open source programming language that promotes rapid program development and readable code
Declaration and use of variables and constants
A VARIABLE in a computer program is a named data store that contains a value that may change during the execution of the program
A CONSTANT in a computer program is a named data store that contains a value that does not change during the execution of the program.
Basic data types
In order for a computer system to process and store data effectively, different kinds of data are formally given different types. This enables:
• data to be stored in an appropriate way, for example, as numbers or characters
• data to be manipulated effectively, for example numbers with mathematical operators and characters with concatenation
• automatic validation in some cases.
Integer
An INTEGER is a positive or negative whole number that can be used with mathematical operators.
Real
A REAL NUMBER is a positive or negative number with a fractional part. Real numbers can be used with mathematical operators. Not all programming languages distinguish between real numbers and integers. JavaScript makes no distinction; Python does with the use of built-in functions.
FirstInteger = int(25)
FirstReal = float(25)
Char
A variable or constant of type CHAR is a single character.
Gender = ‘F’ or Gender = “F”
String
A variable or constant of type STRING is several characters in length
TelephoneNumber = ‘44121654331’
FirstName = ‘Emma’
UnRepeatable = ‘@!&&@@##!’
Boolean
A BOOLEAN variable can have only two values: TRUE or FALSE.
Sequence
Statements are followed in sequence so the order of the statements in a program is important. Assignment statements rely on the variables used in the expression on the right-hand side of the statement all having been given values
FirstNumber = int(input( ‘Enter First Whole Number: ‘))
SecondNumber = int (input( ‘Enter Second Whole Number: ‘))
Sum = FirstNumber + SecondNumber
print (‘The sum equals ‘, Sum)
Selection
It is a very useful technique, allowing data items to be picked out according to given criteria, for example selecting the largest value or the smallest value, selecting items over a certain price, selecting everyone who is male. This is done with the use of if and case statements.
Repetition
Most programming languages support three types of loop:
• a fixed number of repetitions
• an unknown number of repetitions with at least one repetition, as the condition is tested at the end of the loop
• an unknown number of repetitions which may not be completed at all, as the condition is tested at the beginning of the loop.
Totalling
Totalling is used with repetition with the total updated every time the loop is repeated.
ReceiptTotal = ReceiptTotal + CostOfItem
Counting
Counting is used with repetition with the counter increased by 1 every time the loop is repeated
NumberOfItems = NumberOfItems + 1